Create Taxonomy Term Meta in WordPress
Just like post meta data WordPress term meta is additional information stored against a taxonomy term. A single entry in a taxonomy is called term. This post demonstrates how to add term meta to custom taxonomy. We will create a term meta "zipcode" for our property-city taxonomy.
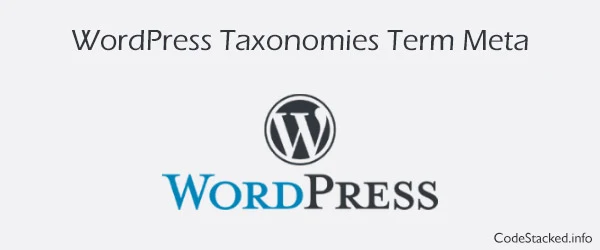
WordPress not only allows us to add custom post types and custom taxonomies but also provides different hooks to extend term form fields. We will use four WordPress action hooks to save term meta for a taxonomy term.
- $taxonomy_add_form_fields hook is to add term meta form fields to new term form.
- $taxonnomy_edit_form_fields hook is to add term meta form fields to edit term form.
- created_$taxonomy hook saves term meta when a new term is created.
- edited_$taxonomy hook saves term meta when an existing term is edited or update.
WordPress hooks can be used like this:
add_action( "$taxonomy_add_form_fields", "function_to_show_fields", 10, 1 ); add_action( "$taxonomy_edit_form_fields", "function_to_show_fields", 10, 2 ); add_action( "created_$taxonomy", "funtion_to_save_fields", 10, 2 ); add_action( "edited_$taxonomy", "funtion_to_save_fields", 10, 2 );
Where $taxonomy is the slug of taxonomy in this post its going to be "city". You need to change it to your taxonomy slug for which you are going create this custom term meta form fields. We will create a separate file terms-meta.php and then include this file in functions.php. We will add mentioned action hooks in our terms-meta.php file to add term form fields. Visit our this post Add Custom Column to Taxonomies Table to learn how to show term meta column in taxonomies table.
Add Term Meta Fields to Term Add and Edit Form
We are going to implement it all at once since the implementation is simple enough. Steps to add term form fields to term form:
- Add action hook $taxonomy_add_form_fields to add term meta field to term add form.
- Add action hook $taxonnomy_edit_form_fields to add term meta field to term edit form.
- Add action hook created_$taxonomy and edited_$taxonomy to save term meta when a term is either created or update.
terms-meta.php
<?php
// Hook to show term field on add term form
add_action('property-city_add_form_fields', 'add_property_city_meta_fields', 10, 2);
function add_property_city_meta_fields() {?>
<div class="form-field term-group selectdiv">
<label class="title-label">
<?php _e('Zipcode', 'text-domain');?>
<span>*</span>
</label>
<input type="number" name="zipcode" class="regular-text" required="required" />
<p class="description"><?php _e('Enter zipcode of city', 'text-domain')?></p>
</div>
<?php
}
// Hook to show term fields on edit taxonomy form
add_action('property-city_edit_form_fields', 'taxonomy_property_city_edit_meta_fields', 10, 2);
function taxonomy_property_city_edit_meta_fields($term, $taxonomy) {
$zipcode = get_term_meta($term->term_id, 'zipcode', true);?>
<tr class="form-field">
<th scope="row" valign="top">
<?php _e('Zipcode', 'text-domain');?>
<span>*</span>
</th>
<td>
<div class="property-city-metabox">
<input type="number" name="zipcode" class="regular-text" value="<?=$zipcode?>" required="required" />
<p class="description"><?php _e('Enter zipcode of city', 'text-domain')?></p>
</div>
</td>
</tr>
<?php
}
// Two hooks with same function to save term meta when term is created/edited
add_action('created_property-city', 'save_taxonomy_property_city_meta', 10, 2);
add_action('edited_property-city', 'edit_property_city_meta', 10, 2);
function edit_property_city_meta( $term_id ) {
if (isset($_POST['zipcode'])) {
update_term_meta($term_id, 'zipcode', esc_html($_POST['zipcode']));
}
}
All done, now all we need to do is include terms-meta.php in our functions.php.
<?php include('terms-meta.php');?>
You can use get_term_meta() function to fetch term meta of a single term:
get_term_meta( $term_id, 'meta_key', true );
Where $term_id is the single term id , "meta_key" is the field name we saved which was "city" used in this post, and last parameter is used for response if set to true it will return a single value.
