Login with Google OAuth in PHP
Filling out online registration forms can be very frustrating for users. Google and many other popular social networks provide login libraries for developers to allow website's user login using their platforms without filling out registration form. We will be using Google OAuth 2.0 to create a Login with Google script.
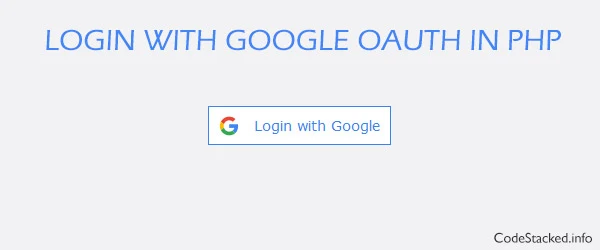
In this post we are going to show you how to login with Google in PHP using Google OAuth API. We will first cover what steps you need to take before diving into PHP side implementation of Google login in PHP. Files we are going to need for this post are:
- constants.php: Contains constants for our application.
- index.php: Contains Google Login Button to allow user to login with google.
- logout.php: Contains the code to revoke access token of current user.
- config.php: Contains the code to call Google Client API.
- style.css: CSS styles for our HTML page and google login button.
Create a Project in Google Cloud Console
First thing you need to do is go to Google Cloud Console and create a new project. Enter a name for project and click create. After you have created your project:
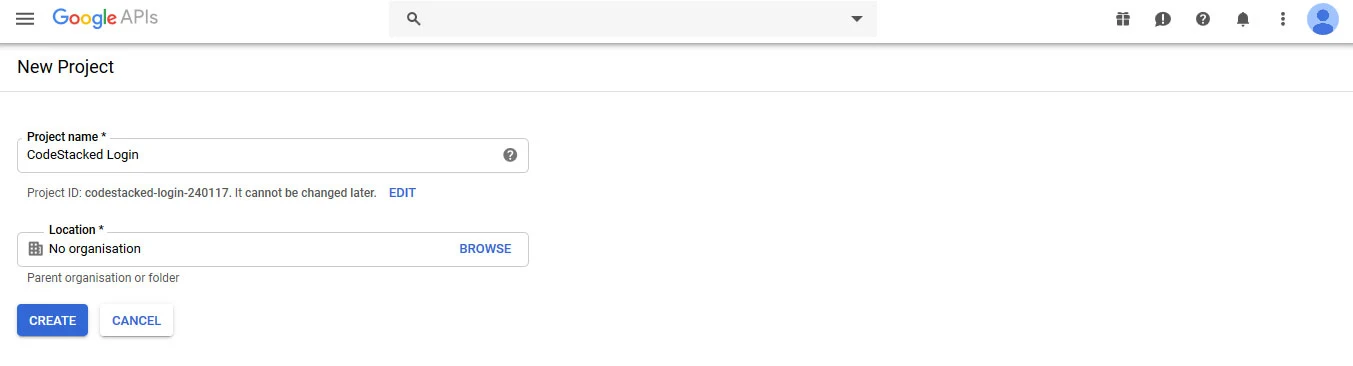
Configure OAuth Consent Screen Settings
After you have created a project in Google API Console you now have to configure consent screen settings.
- Click on credentials on left side menu.
- Click on OAuth Consent Screen tab.
- Enter your application name and upload a logo if needed.
- Select your support email address.
- By default google will add three scopes, profile, email, and openid, you can ignore it at this point or add more scopes.
- Add authorized domains.
- Add application homepage link.
- Add application privacy page link.
- Click save.
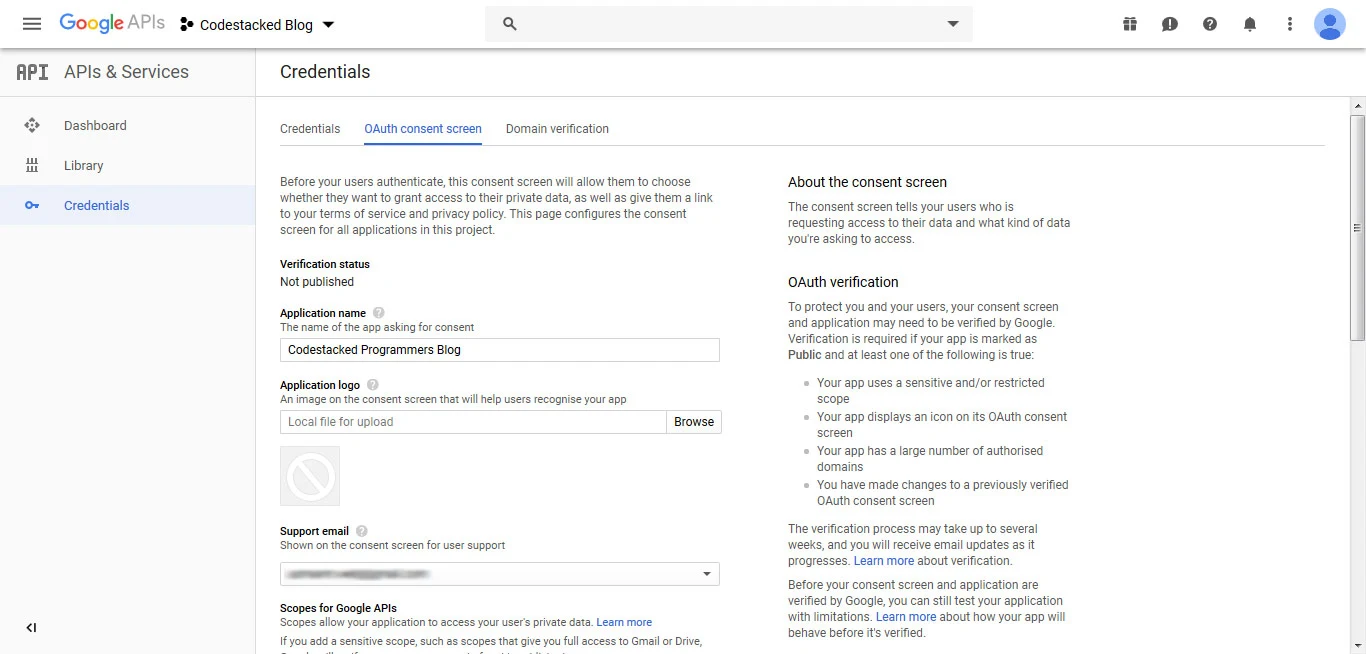
Get OAuth Client ID
Now next phase is to create OAuth ClientID for our web application.
- Click on credentials tab.
- Click on create credentials and select OAuth Client ID from menu.
- Select "Web Application" under Application Type.
- Give your application a name.
- Enter authorized redirect URLs. These redirects are actually the URLs users will be redirected to after they login using their google account.
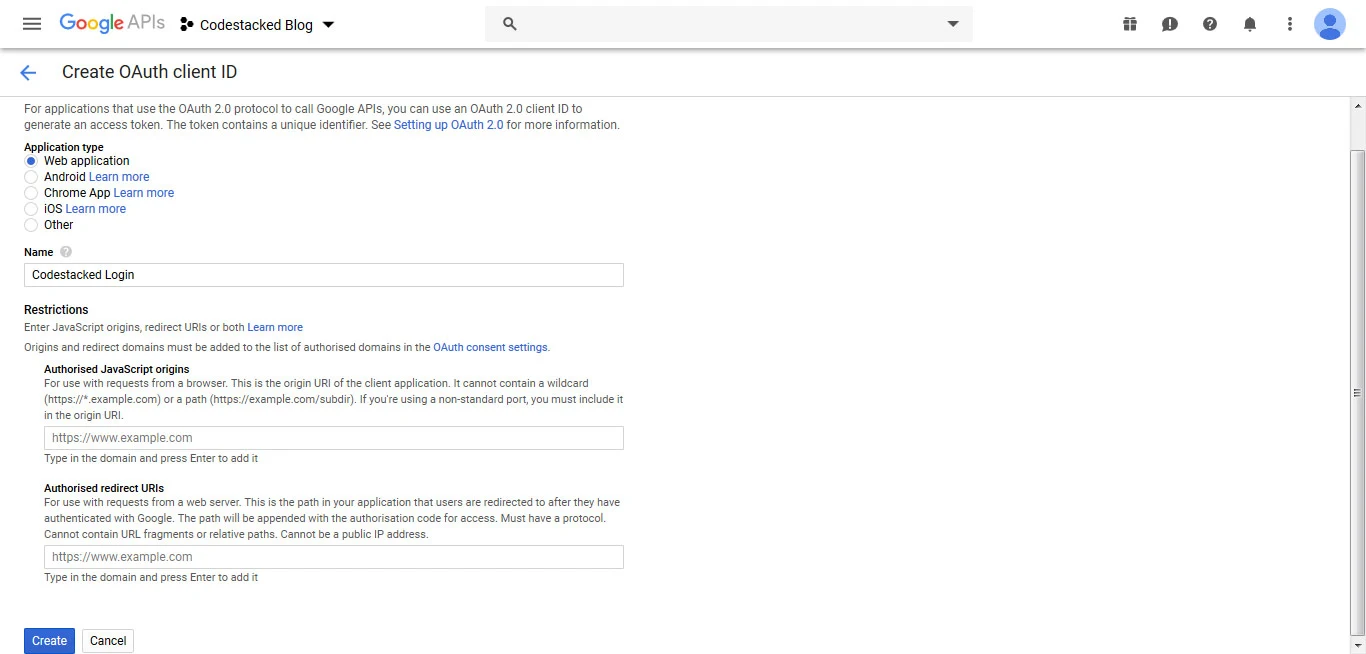
After you have created your OAuth Client ID for web application you will be able to see your client id and client secret. Either download the credentials file or manually copy paste by clicking on OAuth Client ID you will need it later in code.
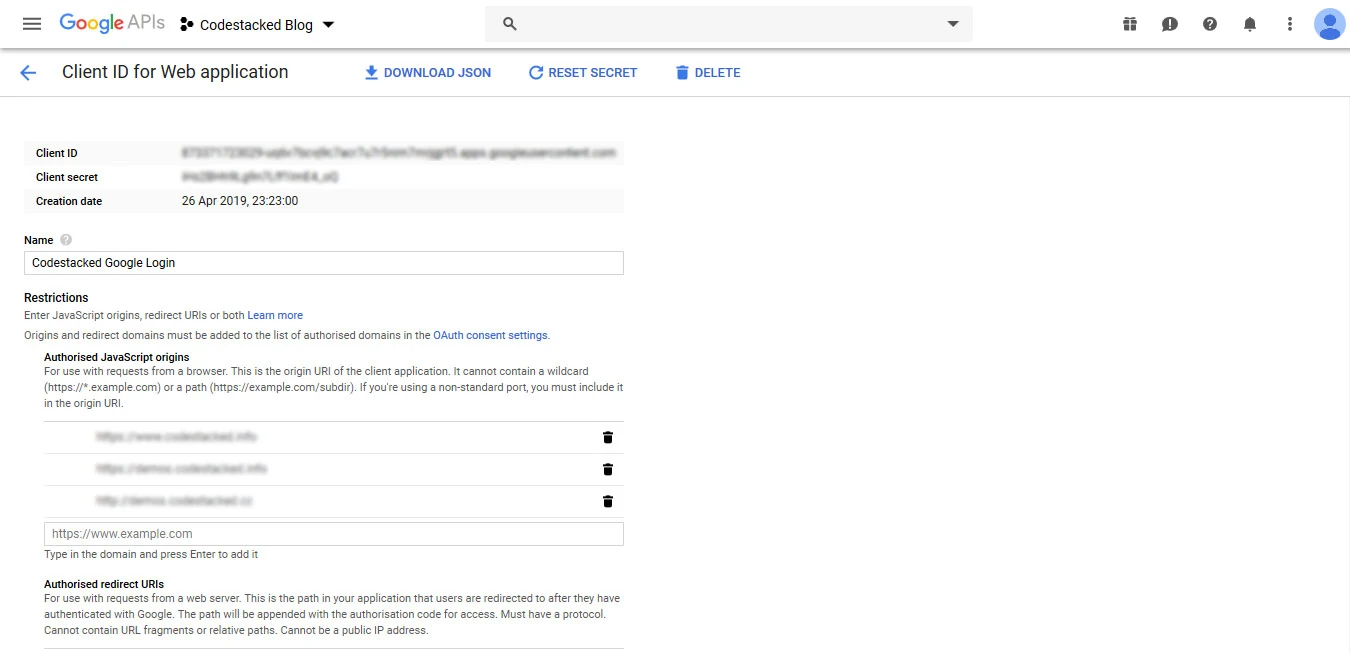
Google API Client Library for PHP
Now before we start coding for our login script for those who will be using it on localhost first. You might need cacert.pem for SSL issuer verification. You can download the fresh cacert.pem from here. Place the cacert.pem file anywhere in your wamp directory and update below two lines in php.ini.
curl.cainfo ="path-to-file-directory/cacert.pem" openssl.capath="path-to-file-directory/cacert.pem"
First download the archive of library from Google API Client PHP Library 2.15.0 from here or download it via composer.
composer require google/apiclient:^2.15.0
Add Constants
Contains the constants for base URL, Application and google client id and secret.
constants.php
<?php
define('BASE_URL', 'https://' . filter_input(INPUT_SERVER, 'SERVER_NAME', FILTER_SANITIZE_URL) . '/');
define('APPLICATION_NAME', 'YOUR_APPLICATION_NAME');
define('CLIENT_ID', 'YOUR_CLIENT_ID');
define('CLIENT_SECRET', 'YOUR_CLIENT_SECRET');
Call Google Client API
Script containg the code to connect to Google Client API.
config.php
<?php
session_start();
// If downloaded via composer
include_once 'vendor/autoload.php';
// If downloaded the archive
include_once 'google-api-php-client-2.2.2/vendor/autoload.php';
include_once 'constants.php';
$client = new Google_Client();
$client->setApplicationName(APPLICATION_NAME);
$client->setClientId(CLIENT_ID);
$client->setClientSecret(CLIENT_SECRET);
$client->setAccessType('offline');
$client->addScope('profile email');
$client->setRedirectUri(BASE_URL.'login-with-google-oauth-in-php');
Add Login with Google Button and Get User Information
Contains the code to show login button allowing user to login with google and user information block if user is already logged in.
- If user is already logged in then set Google OAuth access token from session.
- Get logged in user information from Google OAuth Service and show it on page.
- If user returned to this page from Google Authentication page then get the access token from Google Client API and store it in session.
index.php
<?php
global $client;
include_once 'config.php';
if(isset($_SESSION['access_token'])){
$client->setAccessToken($_SESSION['access_token']);
$service = new Google_Service_Oauth2($client);
$user_info = $service->userinfo->get();
}
if($code = filter_input(INPUT_GET, 'code', FILTER_SANITIZE_SPECIAL_CHARS)){
$client->fetchAccessTokenWithAuthCode($code);
$_SESSION['access_token'] = $client->getAccessToken();
/**
* YOU CAN STORE USER INFORMATION TO DATABASE HERE
*/
header('location: ' . BASE_URL . 'downloads/login-with-google-oauth-in-php');
exit;
}
?>
<!DOCTYPE html>
<html>
<head>
<title>Login with Google OAuth in PHP - Demo</title>
<meta content="text/html; charset=UTF-8" http-equiv="Content-Type"/>
<meta content="width=device-width, initial-scale=1, maximum-scale=1" name="viewport" />
<link rel="stylesheet" href="css/style.css" />
</head>
<body>
<section class="section py-4">
<div class="container">
<?php if(isset($_SESSION['access_token'])){?>
<h3>You are logged in as:</h3>
<div class="user-container">
<div>
<img src="<?=$user_info['picture'];?>"
class="user-thumbnail"
width="96" height="96" alt="" loading="lazy" />
</div>
<div class="user-info">
<div>
<strong><?=$user_info['name']?></strong>
</div>
<div>
<small><?=$user_info['email']?></small>
</div>
<div>
<a href="logout.php">Logout</a>
</div>
</div>
</div>
<?php }else{?>
<a href="<?=$client->createAuthUrl()?>" class="btn-google">Login with Google</a>
<?php } ?>
</div>
</section>
</body>
</html>
Logout with Google API
File which contains the code to logout user and unset the token.
- Unset the access token in session.
- Revoke Google OAuth token.
- Return to the home page or login page again.
Logout.php
<?php
global $client;
include_once 'config.php';
unset($_SESSION['access_token']);
$client->revokeToken();
header('Location:' . BASE_URL . 'login-with-google-oauth-in-php');
Add CSS Styles
Contains the CSS styling for login button and user information block.
style.css
* {
box-sizing: border-box;
text-decoration: none;
}
html,body {
margin: 0;
padding: 0;
}
body {
background-color: #f6f6f6;
font-family: "Segoe UI", "Roboto", "Helvetica", sans-serif;
font-size: 15px;
font-weight: normal;
font-style: normal;
line-height: 1.5;
}
.container {
width: 100%;
max-width: 1140px;
margin-right: auto;
margin-left: auto;
padding-right: 15px;
padding-left: 15px;
}
.py-4 {
padding-top: 1rem;
padding-bottom: 1rem;
}
.user-container {
background-color: #ffffff;
border: 1px solid #dddddd;
display: inline-flex;
gap: 1rem;
padding: 1rem;
}
.user-thumbnail {
max-width: 100%;
height: auto;
display: inline-block;
border-radius: 100%;
}
.btn-google {
background: url("../images/google-icon.png") no-repeat 10px, linear-gradient(90deg, #ffffff 45px, #4081ED 45px);
border: 1px solid #4081ED;
color: #ffffff;
display: inline-block;
padding: 10px 10px 10px 55px;
text-decoration: none;
border-radius: 0.25rem;
}
References:
Using OAuth 2.0 for Web Server Applications